Software engineering predictive search: a complete guide
Learn how to implement predictive search in your software applications. Discover key concepts, optimization techniques, and real-world examples to enhance user experience.
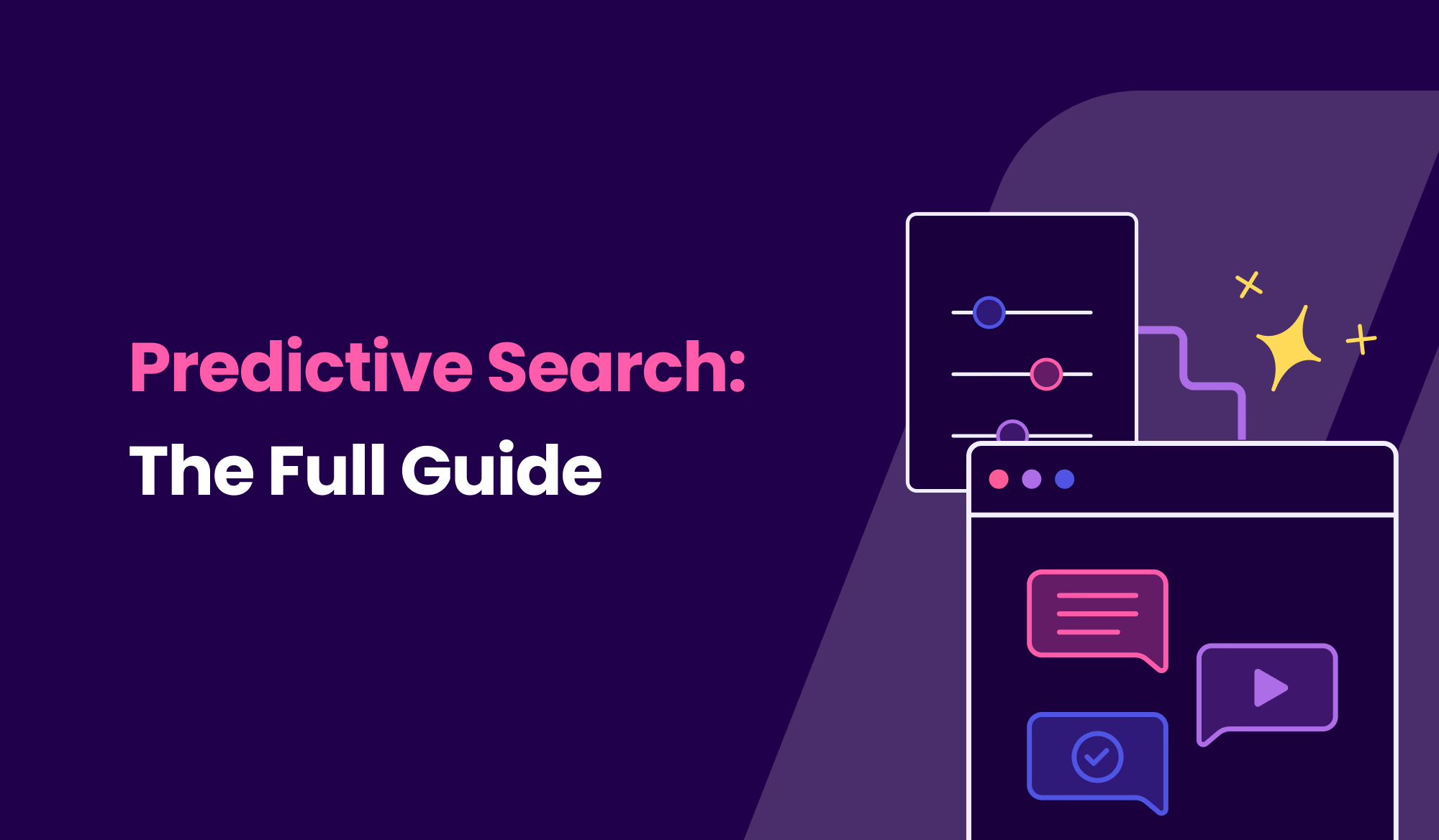
Have you ever noticed how Google suggests exactly what you're looking for before you finish typing? That's predictive search in action. It revolutionizes user interaction with software applications. In this guide, we'll explore implementing predictive search in your applications.
Introduction to Predictive Search
Definition and Importance of Predictive Search
Predictive search is an intelligent system that anticipates user intent and suggests relevant results in real-time as users type. Unlike traditional search methods that wait for a complete query, predictive search processes each keystroke to provide immediate, relevant suggestions.
The importance of predictive search in software engineering is significant. It bridges user intent and content discovery, reducing the time and effort users spend searching. Modern applications leverage predictive search to enhance productivity and streamline workflows.
Software engineering teams implementing predictive search can expect improved user engagement metrics. For instance, e-commerce platforms often see a 25-30% increase in conversion rates with predictive search features. Developer tools report significant reductions in time spent searching for code snippets or documentation.
Predictive Search Enhancing Autocomplete
Traditional autocomplete completes words based on character matching. Predictive search, however, analyzes patterns in user behavior, search history, and contextual data to offer intelligent suggestions.
The personalization aspect of predictive search sets it apart from basic autocomplete. Predictive search creates a more tailored experience by considering factors like previous searches, user location, and trending queries.
For example, when a developer searches for "deploy" in a cloud platform's console, the system prioritizes suggestions based on their commonly used services or recent deployments.
For example, in an e-commerce platform, autocomplete might suggest "laptop bag" when a user types "lap", predictive search might also suggest "computer accessories," "mobile workstation," or specific laptop models based on browsing history and market trends.
Core Concepts and Components
Predictive Models in Software Engineering
Predictive models form the backbone of modern search capabilities. They utilize complex algorithms to analyze user input patterns and generate relevant suggestions.
Businesses can leverage large datasets, with the specific type of data varying depending on the industry and business objectives. It is possible to use various data sources like browsing history, user interactions, and market trends to improve predictive search strategies.
These models process queries through multiple layers of analysis, including string matching, semantic understanding, and historical pattern recognition.
Predictive models often rely on prefix trees (or radix trees) for efficient string matching and retrieval. This structure enables rapid processing of partial queries, allowing the system to generate suggestions with minimal latency. The models continuously learn from user interactions, refining their prediction accuracy over time.
Search Engines and Search Queries
Search engines in predictive systems employ sophisticated indexing mechanisms to aid rapid query processing. Key components include query processors, suggestion generators, and ranking algorithms.
These elements work together to produce and prioritize results based on various relevance factors.
Modern search queries go through steps including tokenization, spell checking, and context analysis. This ensures that even imperfect queries can yield useful suggestions. Balancing immediate results and accuracy often involves using caching mechanisms to optimize frequently requested queries.
Machine Learning and AI Power
Artificial intelligence significantly enhances predictive search through advanced natural language processing and machine learning algorithms.
These technologies help systems understand user intent beyond literal string matching, incorporating semantic understanding and contextual relevance.
AI improves search accuracy by:
- Capturing user intent with natural language understanding
- Adapting ranking algorithms to changing user behavior patterns
- Identifying relationships between different terms through semantic analysis
- Tailoring suggestions based on user profiles and historical interactions
Combining machine learning with traditional search algorithms creates a hybrid approach that delivers both speed and accuracy.
Capabilities Required for Predictive Search
A robust predictive search implementation requires several key capabilities to deliver a seamless user experience. Fast indexing and efficient query processing are foundational, but there's more to consider.
Meilisearch's Approach to Enabling Predictive Search
Meilisearch combines traditional full-text search with AI-powered capabilities. Its architecture enables fast, relevant results and maintains simplicity in implementation.
The integration process focuses on three key areas: data indexing, search configuration, and result optimization. Here's an example of configuring search settings:
// config/search.ts const searchConfig = { settings: { rankingRules: [ 'words', 'typo', 'proximity', 'attribute', 'sort', 'exactness' ], searchableAttributes: [ 'title', 'description', 'category' ], displayedAttributes: [ 'title', 'description', 'category', 'thumbnail' ], // Enable AI-powered search with OpenAI embedder: { type: 'openai', documentTemplate: "Product: {{title}}nDescription: {{description}}" } } }; await searchClient.index('products').updateSettings(searchConfig);
This configuration enables both traditional search capabilities and AI-powered features, creating a hybrid search experience that delivers both speed and relevance. The system handles typos, synonyms, and contextual understanding while maintaining sub-50ms response times.
Ready to supercharge your search experience? Explore Meilisearch Cloud and deliver lightning-fast search results that will keep your users engaged and boost your conversion rates.
Applications and Use Cases
Modern software applications leverage predictive search in numerous ways, from e-commerce platforms to content management systems. Understanding these practical applications helps developers implement the right search strategies for their specific use cases.
App search
App search is a common implementation of predictive search in software applications. Mobile apps benefit particularly due to limited screen space and typing constraints.
For instance, food delivery apps use predictive search to help users find restaurants or dishes quickly, considering factors like previous orders and current location.
Site and media search
Site and media search capabilities have evolved to handle complex content structures. Content management systems and documentation platforms use predictive search to help users navigate large information hierarchies.
For example, technical documentation sites can suggest relevant API endpoints or code examples as developers type their queries.
Check out how Meilisearch powers the discovery of 300,000+ AI models on Hugging Face, demonstrating the power of predictive search in organizing and accessing vast AI resources.
Ecommerce search
E-commerce platforms showcase the potential of predictive search through sophisticated product discovery. Online stores suggest not only product names but also categories, brands, and specific features. This capability extends to handling complex queries like "red summer dress under $50" accurately.
For another compelling example of predictive search in action, explore Meilisearch's case study with Bookshop.org, where they achieved a remarkable 43% increase in search-based purchases by enhancing their book discovery platform.
Enterprise search
Enterprise search presents unique challenges because it handles diverse data sources while maintaining security constraints. Predictive search in enterprise environments must consider user permissions, department-specific terminology, and internal document hierarchies.
For instance, a company's internal tools might suggest different results for the same query based on the user's role and access level.
Techniques and Methodologies
Genetic Programming and Test Models
Genetic programming enhances predictive search capabilities by evolving and refining search algorithms. These techniques mimic natural selection to optimize search patterns and improve relevancy.
The system dynamically generates search algorithm variations. It then evaluates these algorithms by analyzing user interactions. Based on performance metrics, the system selects the most effective algorithm combinations to enhance future search experiences.
Test models developed through genetic programming adapt to changing search patterns. For instance, e-commerce search systems can automatically adjust prediction weights based on seasonal trends, user behavior shifts, and new product introductions. The evolutionary nature of these algorithms ensures relevant search suggestions as user preferences and patterns evolve.
Base Software and Search Base
Establishing a robust search base requires careful consideration of data structure and indexing strategies.
For deeper insights into optimizing search indexing, check our comprehensive guide on best practices for faster indexing.
Typically, it consists of an inverted index system enabling quick lookup of terms while maintaining relationships between search elements. This base structure must support both exact matches and fuzzy searching capabilities.
Integration of predictive search within software frameworks demands:
- Efficient data storage and retrieval mechanisms to handle large volumes of search queries
- Flexible indexing strategies accommodating both structured and unstructured data
- Scalable architecture maintaining performance under increasing load
- Real-time update capabilities to reflect changes in underlying data
The search base must also handle edge cases and special scenarios. For example, with multilingual search capabilities, the base system must account for different character sets, writing systems, and language-specific patterns. This ensures reliable and effective predictive search functionality across diverse use cases and user requirements.
Capabilities
Low latency is crucial for predictive search systems because users expect instant feedback while typing. Modern search implementations must maintain response times under 100ms to feel instantaneous. This speed requirement becomes even more critical when handling complex queries across large datasets.
Several optimization techniques help achieve these performance goals. Intelligent caching strategies store frequently accessed results and partial queries, significantly reducing database load and response times for common searches. Here's how response times typically break down:
Operation | Target Latency | Optimization Method |
---|---|---|
Query Processing | < 20ms | In-memory processing |
Index Lookup | < 30ms | Cached indices |
Result Ranking | < 30ms | Pre-computed scores |
Network Transfer | < 20ms | Edge caching |
Ensuring reliability involves implementing robust error handling and failover mechanisms. Search systems must gracefully handle scenarios like network issues, invalid queries, or backend service disruptions. Implementing circuit breakers and fallback options helps maintain service availability.
Performance monitoring is vital for maintaining optimal search functionality. Modern search implementations use real-time metrics tracking to identify bottlenecks and optimization opportunities.
Key performance benchmarks for predictive search systems include:
- Query response time: 95th percentile under 200ms
- Suggestion relevancy: Minimum 80% accuracy rate for top 5 suggestions
- System throughput: Ability to handle 1000+ queries per second per node
- Cache hit ratio: At least 85% cache effectiveness
Meilisearch Cloud includes built-in monitoring tools that enable real-time tracking of search performance metrics.
The architecture should scale horizontally to handle increasing load while maintaining performance. This typically involves:
- Distributed index sharding for better resource utilization
- Load balancing across multiple search nodes
- Asynchronous index updates to prevent blocking operations
- Smart query routing based on user location and server load
Reliability also extends to data consistency and accuracy. Search systems must handle index updates and real-time data changes without compromising search quality or availability. This often requires sophisticated versioning and synchronization mechanisms to ensure users always see the most relevant and up-to-date results.
Future Directions and Innovations
As predictive search technology continues to evolve, several emerging trends and innovations are reshaping the future of search systems.
Integration with Advanced Technologies
The future of predictive search is being shaped by advancements in artificial intelligence and machine learning technologies. Large language models (LLMs) are transforming how search systems understand and process user queries. These models can interpret natural language with high accuracy, enabling more conversational and context-aware search experiences.
Integration with advanced technologies is already showing promising results. Some systems are experimenting with multimodal search capabilities, processing text, images, and voice inputs simultaneously. This enables more intuitive search interfaces that match how humans naturally communicate.
Trends in Predictive Search
LLMs are revolutionizing predictive search capabilities by fundamentally transforming how we interact with search engines. These sophisticated systems can now comprehend complex queries and generate natural, contextually appropriate suggestions that feel remarkably human-like.
Modern search systems now interpret both technical and informal language, moving beyond pattern matching to understand the true meaning behind user queries.
This advancement has enabled several crucial improvements in search functionality:
- More precise interpretation of user queries
- Sophisticated handling of ambiguous searches
- Enhanced recognition of specialized terminology
- Intelligent, context-aware result ranking
These innovations are already being implemented in various forms. Some search engines use hybrid approaches that combine traditional search algorithms with AI-powered semantic understanding. This allows them to maintain the speed and reliability of conventional search while leveraging advanced AI capabilities.
Predictive Search is the Future Present
Predictive search has evolved from a convenient feature to an essential component of modern software applications.
Successful implementation requires careful consideration of architecture, performance optimization, and user experience design.
The integration of advanced AI technologies and mobile-first approaches will continue to push the boundaries of what's possible in predictive search. This makes it an exciting area for continued innovation and development.
Unlock advanced search potential with customizable relevancy, typo tolerance, and more. Enhance Your Search Strategy with Meilisearch Cloud's powerful search capabilities.