Fuzzy search: a comprehensive guide to implementation
Learn how to implement fuzzy search to handle typos and misspellings in your applications. Get practical code examples and best practices for better UX.
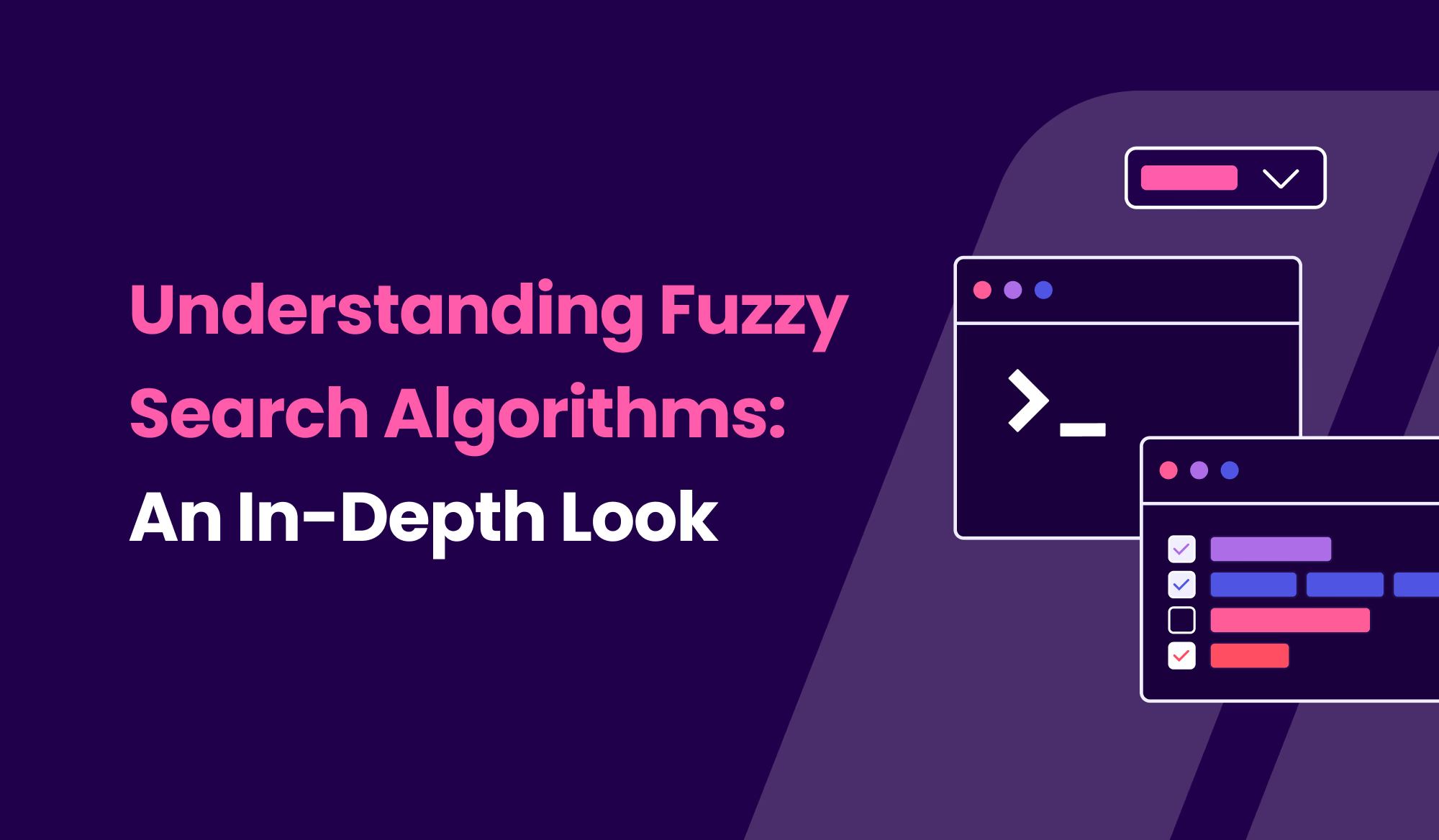
Ever struggled to find exactly what you need because of a small typo or misspelling? Imagine losing potential customers or watching users abandon your platform in frustration.
Fuzzy search is your secret weapon to transform user experience and turn near-misses into successful discoveries.
This guide will explore how fuzzy search works, explain why it has become essential for customer-centric applications, and demonstrate its practical implementation. By prioritizing seamless, intuitive interactions, fuzzy search transforms the way users find and access information.
What is fuzzy search?
Fuzzy search is a powerful search technique that finds matches even when users make mistakes in their queries. Unlike traditional exact-match searching, fuzzy search understands and accommodates human error. This makes it an invaluable tool in modern applications.
Fuzzy search meaning
Fuzzy search works like a forgiving librarian. It finds approximate matches rather than requiring perfect input. When you type "restarant" into a search box, fuzzy search knows you probably meant "restaurant" and shows you relevant results anyway.
The key difference between fuzzy search and exact search lies in their approach to matching. Traditional exact search is like a strict teacher who marks answers as either right or wrong. The input must match the stored data perfectly. Fuzzy search, however, uses sophisticated algorithms to measure how similar strings are to each other. This allows for variations and mistakes.
Fuzzy search solves several common problems in data retrieval:
- Handles typos, misspellings, and alternate spellings
- Matches abbreviations
- Handles phonetic variations
- Accounts for regional spelling differences like "color" versus "colour"
Importance of fuzzy search
User experience dramatically improves with fuzzy search implementation. Instead of frustrating "no results found" messages, users get helpful suggestions and relevant results. This reduction in search friction leads to higher user satisfaction and increased engagement.
Implementing fuzzy search offers significant business benefits. Effective on-site search can nearly double conversion rates, with advanced search capabilities boosting desktop conversions by 50%. By implementing intelligent search functionality, businesses can improve user experience and drive revenue growth.
Major companies have seen significant benefits from implementing fuzzy search. Amazon's search system helps customers find products even with spelling mistakes. Google's search engine famously asks "Did you mean...?" when it detects potential errors. These implementations have become so successful that users now expect this level of search intelligence in all their applications.
How fuzzy search works
Understanding how fuzzy search operates requires diving into its core algorithms and mechanisms for handling typos. These components create a robust search system that can interpret and correct user input errors.
Core algorithms
The Levenshtein distance algorithm serves as the foundation for many fuzzy search implementations. It calculates the minimum number of single-character edits needed to change one word into another. For example, transforming "kitten" to "sitting" requires three edits: substitute 'k' for 's', substitute 'e' for 'i', and insert 'g' at the end.
Hamming distance offers a simpler approach by counting positions where corresponding characters differ between two strings of equal length. While more limited than Levenshtein, it detects single-character mutations in strings of the same length. For instance, "color" and "colour" have a Hamming distance of 1.
The Damerau-Levenshtein distance builds on the basic Levenshtein algorithm by adding transposition of adjacent characters as a valid operation. This makes it especially effective at catching common typing errors where users accidentally swap letters, like typing "teh" instead of "the."
Each algorithm has trade-offs:
- Levenshtein provides comprehensive error detection but can be computationally expensive.
- Hamming distance offers faster processing but only works with strings of equal length.
- Damerau-Levenshtein balances accuracy and performance but requires more complex implementation.
Typo tolerance mechanisms
A typo occurs when users make unintentional mistakes while entering text. These can include:
- Character substitutions (typing 'a' instead of 's')
- Omissions (leaving out a letter)
- Additions (adding an extra letter)
- Transpositions (swapping adjacent letters)
Spelling distance calculations help determine how different two strings are from each other. The lower the distance score, the more similar the strings. For example, "apple" and "appl" have a distance of 1 (one deletion), while "apple" and "aple" also have a distance of 1 (one deletion).
Consider a real-world example: searching for "Mississippi" in a database. A user might type "Missisippi" (missing one 's'). A well-configured fuzzy search system would calculate the spelling distance, recognize the high similarity between the strings, and return results for the correct spelling.
Search results are affected by how tolerant the system is of typos. Setting the tolerance too high might return too many irrelevant results, while setting it too low might miss valid matches. Most systems use a threshold based on the length of the search term, allowing more typos for longer words.
Step-by-step implementation guide
Implementing fuzzy search doesn't have to be complicated. Let's walk through a practical implementation using TypeScript and Fuse.js, which offers powerful fuzzy searching out of the box.
Basic implementation steps
First, set up a basic fuzzy search system. Here's how to create a simple search function that handles typos and misspellings:
import Fuse from 'fuse.js'; interface Product { name: string; description: string; price: number; } // Sample product data const products: Product[] = [ { name: 'iPhone 13', description: 'Latest Apple smartphone', price: 999 }, { name: 'Samsung Galaxy', description: 'Android flagship phone', price: 899 }, ]; // Configure Fuse options const options = { keys: ['name', 'description'], // Fields to search threshold: 0.3, // Lower means stricter matching minMatchCharLength: 3 // Minimum characters that must match }; // Initialize Fuse const fuse = new Fuse(products, options); // Search function function searchProducts(query: string): Product[] { return fuse.search(query).map(result => result.item); }
The code sets up a Fuse.js fuzzy search with low threshold and minimum match length, enabling flexible product searching. This configuration allows users to find products through partial or approximate matches, even with typing errors or incomplete search terms.
Real world example scenario
Now, expand the implementation for a more realistic e-commerce search scenario:
interface SearchResult { items: Product[]; didYouMean?: string; totalResults: number; } class ProductSearch { private fuse: Fuse<Product>; constructor(products: Product[]) { this.fuse = new Fuse(products, { keys: [ { name: 'name', weight: 0.7 }, { name: 'description', weight: 0.3 } ], threshold: 0.4, distance: 100 }); } search(query: string): SearchResult { const results = this.fuse.search(query); return { items: results.map(r => r.item), totalResults: results.length }; } } // Usage example const searchEngine = new ProductSearch(products); const results = searchEngine.search('iphone');
The code snippet demonstrates a ProductSearch
class that implements fuzzy search functionality. The search
method takes a query string, performs a fuzzy search on a collection of products, and returns search results including matched items, a "did you mean" suggestion, and the total number of results.
Common Pitfalls During Implementation
When implementing fuzzy search, developers often face several challenges. The threshold setting is tricky – set it too low and you'll miss valid matches, set it too high and you'll get irrelevant results. Start with a threshold around 0.3-0.4 and adjust based on your needs.
Memory usage can also be a concern with large datasets. To address this, implement pagination and limit the result number.
Implementation using Meilisearch typo tolerance
Meilisearch offers a robust and configurable typo tolerance system, making it straightforward to implement fuzzy search. Let's explore how to set up and fine-tune Meilisearch's typo tolerance features for optimal search results.
Basic configuration
Setting up typo tolerance in Meilisearch starts with basic configuration options. Here's how to customize the fundamental settings:
import { MeiliSearch } from 'meilisearch' const client = new MeiliSearch({ host: 'http://localhost:7700', apiKey: 'masterKey' }) // Configure typo tolerance settings await client.index('products').updateTypoTolerance({ enabled: true, minWordSizeForTypos: { oneTypo: 5, // Allow one typo for words >= 5 characters twoTypos: 9 // Allow two typos for words >= 9 characters } })
Advanced typo settings
Meilisearch allows fine-grained control over typo tolerance for specific words or attributes. This is useful for dealing with brand names or technical terms:
// Disable typo tolerance for specific words await client.index('products').updateTypoTolerance({ disableOnWords: ['iphone', 'xbox', 'playstation'], disableOnAttributes: ['brand_name', 'sku'] }) // Configure search settings const searchConfig = { limit: 20, attributesToHighlight: ['name', 'description'], typoTolerance: { enabled: true, minWordLength: 4 } } // Perform search with configured settings const results = await client.index('products') .search('iphone', searchConfig)
Custom rules and exceptions
Sometimes, you need to handle special cases where standard typo tolerance rules don't fit. Meilisearch provides ways to implement custom rules, (like dictionnaries and synonyms):
// Create custom dictionary for common misspellings await client.index('products').updateDictionary({ synonyms: { 'fone': ['phone'], 'laptop': ['labtop', 'loptop'], 'wireless': ['wirelss', 'wireles'] } }) // Configure word relationships await client.index('products').updateSettings({ stopWords: ['the', 'a', 'an'], rankingRules: [ 'words', 'typo', 'proximity', 'attribute', 'sort', 'exactness' ] })
These implementations provide a solid foundation for building a search system that gracefully handles user typos while maintaining relevant results. Remember to adjust these settings based on your specific use case and user feedback.
Want to see fuzzy search in action without the hassle of implementation? Check out Meilisearch Cloud, which offers powerful, out-of-the-box fuzzy search capabilities for your projects.
Implementing fuzzy search in SQL
SQL databases provide built-in functions for fuzzy matching. Trigram similarity is a powerful technique for fuzzy matching, especially with the PostgreSQL pg_trgm
extension:
-- Trigram similarity example CREATE EXTENSION pg_trgm; SELECT * FROM users WHERE similarity(name, 'John') > 0.3;
For more precise matching, you can also use Levenshtein distance to find similar strings:
-- Create a basic fuzzy search function using Levenshtein distance SELECT name, description FROM products WHERE levenshtein(name, 'iphone') <= 2;
You can even combine different fuzzy matching techniques for more comprehensive search results:
-- Combine trigram and distance-based matching SELECT name, description FROM products WHERE similarity(name, 'iphone') > 0.3 OR levenshtein(name, 'iphone') <= 2;
For better performance, create indexes on frequently searched columns and use materialized views for large datasets.
While SQL databases offer basic fuzzy search capabilities, they come with significant limitations for advanced search requirements. As explored in a detailed analysis of Postgres full-text search constraints, traditional databases struggle with complex search scenarios. These limitations include:
-
Complex configuration requirements for advanced search features
-
Performance degradation with large datasets
-
Limited support for nuanced search capabilities like typo tolerance and faceted search
Use cases and applications
Fuzzy search has become an essential feature in various industries, transforming how users interact with search interfaces. Its applications continue to grow and evolve, from e-commerce to content management.
Typical scenarios for fuzzy search
E-commerce platforms are a compelling use case for fuzzy search. When customers search for "Nike snekers" instead of "Nike sneakers," they still need to find the right products. Major retailers like Amazon and Walmart use sophisticated fuzzy search systems to handle common misspellings and variations in product names.
Want to see how a real-world bookstore increased search-based purchases by 43%? Check out the full Bookshop.org case study to learn how they transformed their search experience with Meilisearch.
Content management systems also benefit significantly from fuzzy search. On a news website, journalists need to quickly find articles about "Barack Obama" even if they type "Barak Obama." These systems often implement fuzzy search with different tolerance levels based on content type.
User directories and CMS showcase another powerful application. HR systems often search through employee databases where names have multiple valid spellings. For example, "Catherine," "Katherine," and "Kathryn" should all return relevant results when looking for an employee.
Success stories from real implementations demonstrate the impact of fuzzy search. Bigstock's case study reveals how a fuzzy auto-suggest algorithm transformed their stock image website's search experience. By correcting misspellings and suggesting results based on intended meanings, they achieved:
- A 9.6% increase in users selecting suggested results
- A 6.52% rise in images added to the cart
- A 3.2% boost in image downloads
Best practices and optimization
Implementing fuzzy search is just the first step. Optimizing its performance and ensuring it scales effectively is crucial for maintaining a responsive application. Let's explore key strategies for maximizing fuzzy search efficiency while maintaining accuracy.
Performance optimization techniques
Indexing plays a vital role in fuzzy search performance. A well-designed index can dramatically reduce search times and improve response rates. Think of it like a book's index - it helps you find content without scanning every page. Modern applications typically implement three key optimization strategies:
Pre-computation helps reduce runtime calculations. By processing and storing common variations of search terms in advance, systems can respond more quickly to user queries. For example, storing both "color" and "colour" variations in the index eliminates the need to calculate these matches during runtime.
Query optimization techniques improve search efficiency:
- Limit the search scope to relevant fields
- Use prefix indexing for faster partial matches
- Implement caching for frequent searches
- Set appropriate threshold values to balance accuracy and speed
Memory management becomes crucial as datasets grow. Implementing a smart caching strategy can significantly improve performance. For example, caching recent search results can reduce server load by up to 40% in high-traffic applications.
Want to dive deeper into optimizing search performance? Check out our best practices for faster indexing to supercharge your search implementation
Scalability considerations
Cloud-based solutions offer superior scalability for fuzzy search implementations.
Meilisearch can handle millions of records while maintaining fast response times. It automatically manages resource allocation and scaling based on demand.
When choosing between cloud and on-premise solutions, consider these factors:
- Data volume and growth rate
- Search query frequency
- Response time requirements
- Budget constraints
- Data privacy requirements
Performance at scale requires careful attention to database design and query optimization. Effective techniques include:
- Sharding large datasets across multiple servers
- Implementing load balancing for high-traffic scenarios
- Using asynchronous processing for complex searches
- Regular monitoring and performance tuning
Scaling fuzzy search isn't just about handling more data - it's about maintaining search quality and speed as your application grows. Regular performance testing and monitoring help ensure your system continues to meet user expectations.
Ready to supercharge your search experience? Explore Meilisearch Cloud and deliver lightning-fast search results that will keep your users engaged and boost your conversion rates.
Common challenges and troubleshooting
Every fuzzy search implementation faces unique challenges. Understanding these common hurdles and knowing how to address them helps create more robust and reliable search solutions. Let's explore the main challenges and their solutions.
Accuracy vs performance trade-offs
Balancing search accuracy and speed often feels like walking a tightrope. Emphasizing accuracy can slow down your search, while prioritizing speed might lead to irrelevant results. Most successful implementations start with a baseline configuration and adjust based on user feedback.
Practical approaches to balance accuracy and performance:
- Implement tiered searching that starts with exact matches before falling back to fuzzy matching
- Use different threshold settings for different field types
- Adjust match sensitivity based on query length
- Cache frequently searched terms and their results
For example, an e-commerce site might use stricter matching for product codes but allow more flexibility when searching product descriptions. This approach maintains accuracy where it matters most while providing a forgiving search experience for general queries.
Error handling strategies
Dealing with incomplete or noisy data presents unique challenges in fuzzy search implementations. Common issues include missing fields, inconsistent formatting, and special characters. A robust error handling strategy helps maintain search quality despite these data imperfections.
Successful systems manage these challenges by:
- Implementing data cleaning and normalization pipelines
- Using fallback search strategies when primary methods fail
- Monitoring and logging search failures for continuous improvement
- Providing meaningful feedback to users when searches yield no results
Testing fuzzy search configurations requires a systematic approach. Create a comprehensive test suite that includes:
- Edge cases with special characters and accents
- Common misspellings and typos
- Queries in different languages
- Boundary conditions for match thresholds
Regular validation of search results helps identify areas needing adjustment. Track metrics like false positive rates and user satisfaction to guide configuration updates. Remember that fuzzy search is not a set-it-and-forget-it feature - it requires ongoing monitoring and refinement to maintain optimal performance.
Fuzzy search enhances information retrieval by accommodating human errors and improving search accuracy across platforms. Its applications span from e-commerce to in-app search, making it increasingly essential for businesses seeking intuitive and effective systems that drive user engagement.
Want to take your search functionality to the next level? Learn how predictive search can enhance user experience and help users find exactly what they're looking for faster.
FAQs
Understanding the nuances between different search techniques helps developers make informed decisions about their implementations. Here are answers to the most common questions about fuzzy search technology.
What is the difference between fuzzy search and wildcard search?
Fuzzy search uses algorithms to find approximate matches based on similarity. Wildcard search looks for exact patterns using placeholder characters. Think of fuzzy search as a smart friend who understands what you mean, while wildcard search is more like a pattern-matching robot.
For example:
- Wildcard search: "cat*" matches "category" and "cathedral" but not "kat."
- Fuzzy search: recognizes "kat" as a likely match for "cat" based on string similarity metrics like Levenshtein distance.
This fundamental difference makes fuzzy search more suitable for handling human error and natural language variations.
How does fuzzy search compare with semantic search?
Semantic search represents a more advanced approach to understanding user intent. Fuzzy search focuses on character-level similarities, while semantic search considers the meaning and context of words.
Consider these differences:
- Fuzzy search might match "dog" with "fog" due to character similarity.
- Semantic search would connect "dog" with "puppy" due to meaning.
- Fuzzy search excels at handling typos and misspellings.
- Semantic search better understands synonyms and related concepts.
Want to dive deeper into the nuances of search technologies? Check out our in-depth comparison of full-text and vector search.
What is fuzzy search in SQL?
SQL implementations of fuzzy search rely on functions or extensions that calculate string similarities. Most use variations of the Levenshtein distance algorithm or similar matching techniques.
A basic SQL fuzzy search might look like this:
SELECT * FROM products WHERE similarity(product_name, 'searchterm') > 0.4;
Common SQL fuzzy search methods include:
- Using SOUNDEX or DIFFERENCE functions
- Implementing Levenshtein distance calculations
- Creating custom similarity functions
- Utilizing full-text search capabilities
How do I choose the right fuzzy search algorithm?
Choosing the appropriate fuzzy search algorithm depends on your specific use case and requirements. Consider factors like data type, search volume, and performance needs.
Key selection criteria include:
- Dataset size and growth expectations
- Required search speed and response times
- Accuracy requirements for your use case
- Available computational resources
- Language and character set support needs
Different algorithms excel in different scenarios:
- For name matching, Jaro-Winkler might be ideal.
- For general text searching, Levenshtein distance could work better.
Testing multiple algorithms with your actual data provides the best insights for making this decision. Implementing fuzzy search is no longer a luxury but a necessity for modern applications that prioritize user experience. Whether you're building an e-commerce platform, a document management system, or a simple search interface, handling typos and variations can significantly improve user satisfaction and engagement.